在第一个MVC教程章中,我们学会了如何在MVC控制器和视图进行交互。在本教程中,我们将向前更进一步,学习如何使用模型创建高级应用程序来创建,编辑,删除用户,在我们的应用程序中查看列表。
下面是用来创建高级MVC应用程序的步骤
第1步:选择 File->New->Project->ASP.NET MVC Web应用. 并命名为:AdvancedMVCApplication. 单击确定。在接下来的窗口中,选择模板作为互联网应用程序和视图引擎为Razor。注意,我们这个时候使用的是模板,而不是一个空的应用程序。
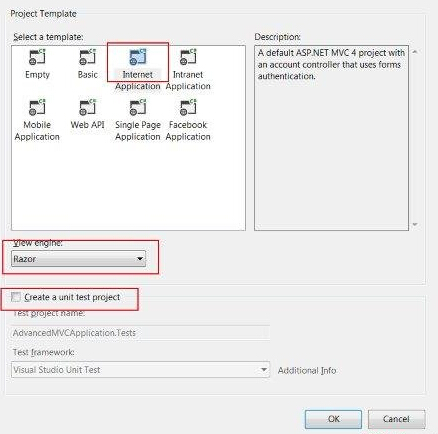
这将创建一个新的解决方案的项目,如下图所示。由于我们使用的是默认的ASP.NET主题,它带有样本视图,控制器,模型和其他文件。
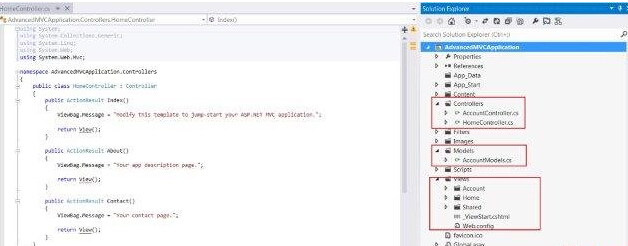
构建解决方案,并运行应用程序,看看它的默认输出,如下:
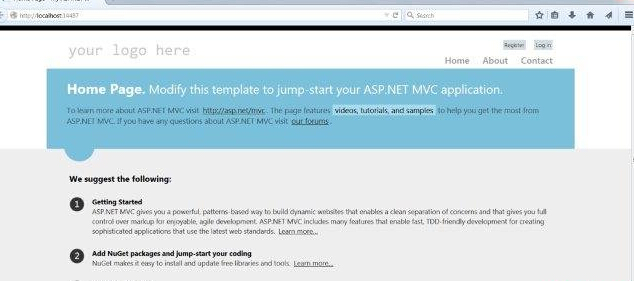
第2步:我们将增加一个新的模式,将定义的用户数据结构。右键单击Models文件夹,然后点击 Add->Class. 命名为UserModel,然后单击Add。
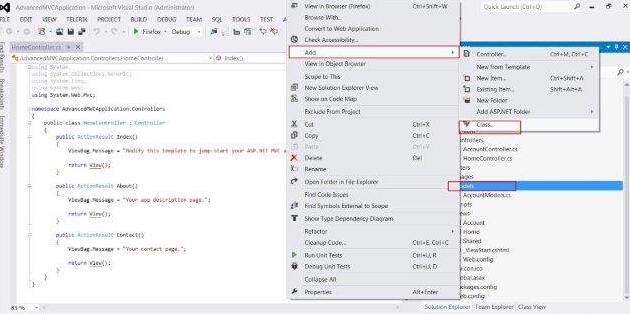
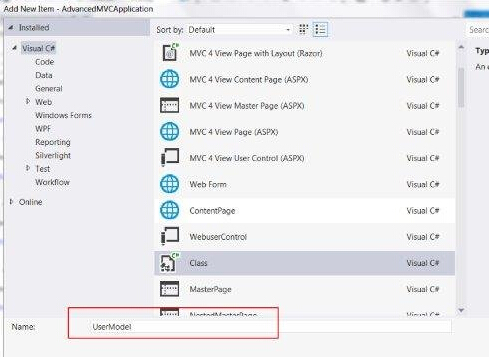
第3步:现在将以下代码复制到新创建的UserModel.cs:
using System;
using System.ComponentModel;
using System.ComponentModel.DataAnnotations;
using System.Web.Mvc.Html;
namespace AdvancedMVCApplication.Models
{
public class UserModels
{
[Required]
public int Id { get; set; }
[DisplayName("First Name")]
[Required(ErrorMessage = "First name is required")]
public string FirstName { get; set; }
[Required]
public string LastName { get; set; }
public string Address { get; set; }
[Required]
[StringLength(50)]
public string Email { get; set; }
[DataType(DataType.Date)]
public DateTime DOB { get; set; }
[Range(100,1000000)]
public decimal Salary { get; set; }
}
} |
在上面的代码中,我们指定的用户模型验证所有的参数,其数据类型和如所需的字段和长度。
第4步:现在,我们有用户模型准备保存数据,现在创建一个类文件Users.cs 其中将包含用于查看用户,添加,编辑和删除用户的方法。右键单击模型,然后单击 Add->Class. 命名为:Users. 这将创建users.cs类在Models内部。
复制下面的代码到users.cs类。
using System;
using System.Collections.Generic;
using System.EnterpriseServices;
namespace AdvancedMVCApplication.Models
{
public class Users
{
public List UserList = new List();
//action to get user details
public UserModels GetUser(int id)
{
UserModels usrMdl = null;
foreach (UserModels um in UserList)
if (um.Id == id)
usrMdl = um;
return usrMdl;
}
//action to create new user
public void CreateUser(UserModels userModel)
{
UserList.Add(userModel);
}
//action to udpate existing user
public void UpdateUser(UserModels userModel)
{
foreach (UserModels usrlst in UserList)
{
if (usrlst.Id == userModel.Id)
{
usrlst.Address = userModel.Address;
usrlst.DOB = userModel.DOB;
usrlst.Email = userModel.Email;
usrlst.FirstName = userModel.FirstName;
usrlst.LastName = userModel.LastName;
usrlst.Salary = userModel.Salary;
break;
}
}
}
//action to delete exising user
public void DeleteUser(UserModels userModel)
{
foreach (UserModels usrlst in UserList)
{
if (usrlst.Id == userModel.Id)
{
UserList.Remove(usrlst);
break;
}
}
}
}
} |
第5步:一旦我们有UserModel.cs和Users.cs,将增加浏览模型查看,添加,编辑和删除用户。首先,让我们创建一个视图用来创建用户。右键单击Views文件夹,然后点击 Add->View.
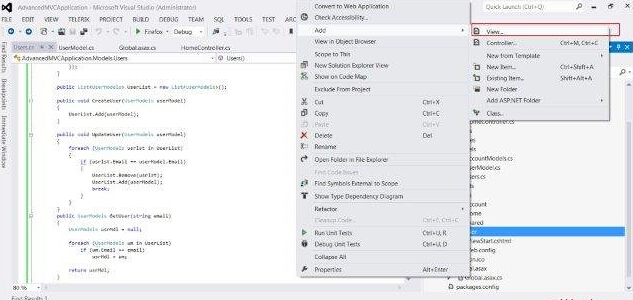
在接下来的窗口中,选择视图名称为UserAdd,视图引擎为Razor,并选择创建一个强类型的视图复选框。
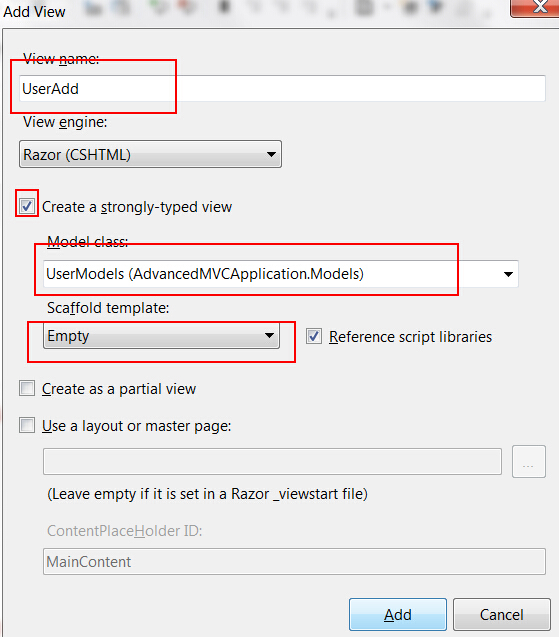
单击添加。在默认情况下这将创建下列CSHML代码,如下所示:
@model AdvancedMVCApplication.Models.UserModels
@{
ViewBag.Title = "UserAdd";
}
<h2>UserAdd</h2>
@using (Html.BeginForm()) {
@Html.ValidationSummary(true)
<fieldset>
<legend>UserModels</legend>
<div class="editor-label">
@Html.LabelFor(model => model.FirstName)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.FirstName)
@Html.ValidationMessageFor(model => model.FirstName)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.LastName)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.LastName)
@Html.ValidationMessageFor(model => model.LastName)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Address)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Address)
@Html.ValidationMessageFor(model => model.Address)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Email)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Email)
@Html.ValidationMessageFor(model => model.Email)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.DOB)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.DOB)
@Html.ValidationMessageFor(model => model.DOB)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Salary)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Salary)
@Html.ValidationMessageFor(model => model.Salary)
</div>
<p>
<input type="submit" value="Create" />
</p>
</fieldset>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
} |
正如所看到的,这个视图包含字段的所有属性信息的验证消息,标签等,此视图在我们最终的应用程序看起来像这样:
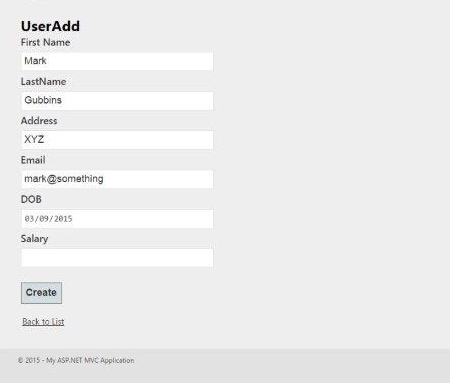
类似UserAdd,,现在我们将增加如下四个视图代码:
Index.cshtml
该视图将显示所有存在于我们的系统中的用户在Index页面上。
@model IEnumerable<AdvancedMVCApplication.Models.UserModels>
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<p>
@Html.ActionLink("Create New", "UserAdd")
</p>
<table>
<tr>
<th>
@Html.DisplayNameFor(model => model.FirstName)
</th>
<th>
@Html.DisplayNameFor(model => model.LastName)
</th>
<th>
@Html.DisplayNameFor(model => model.Address)
</th>
<th>
@Html.DisplayNameFor(model => model.Email)
</th>
<th>
@Html.DisplayNameFor(model => model.DOB)
</th>
<th>
@Html.DisplayNameFor(model => model.Salary)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.FirstName)
</td>
<td>
@Html.DisplayFor(modelItem => item.LastName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Address)
</td>
<td>
@Html.DisplayFor(modelItem => item.Email)
</td>
<td>
@Html.DisplayFor(modelItem => item.DOB)
</td>
<td>
@Html.DisplayFor(modelItem => item.Salary)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.Id }) |
@Html.ActionLink("Details", "Details", new { id=item.Id }) |
@Html.ActionLink("Delete", "Delete", new { id=item.Id })
</td>
</tr>
}
</table> |
此视图在我们最终的应用程序看起来如下:
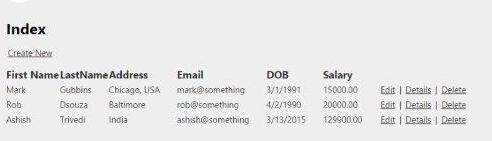
Details.cshtml:
此视图将显示特定用户的详细信息,当我们点击用户记录。
@model AdvancedMVCApplication.Models.UserModels
@{
ViewBag.Title = "Details";
}
<h2>Details</h2>
<fieldset>
<legend>UserModels</legend>
<div class="display-label">
@Html.DisplayNameFor(model => model.FirstName)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.FirstName)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.LastName)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.LastName)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Address)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Address)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Email)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Email)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.DOB)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.DOB)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Salary)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Salary)
</div>
</fieldset>
<p>
@Html.ActionLink("Edit", "Edit", new { id=Model.Id }) |
@Html.ActionLink("Back to List", "Index")
</p> |
此视图在我们最终的应用程序看起来像这样:
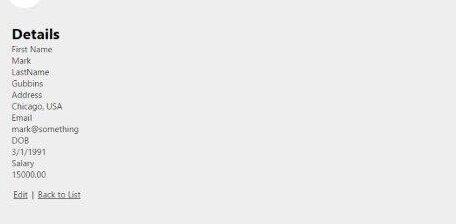
Edit.cshtml:
这视图将显示现有用户的详细信息的编辑表单。
@model AdvancedMVCApplication.Models.UserModels
@{
ViewBag.Title = "Edit";
}
<h2>Edit</h2>
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
@Html.ValidationSummary(true)
<fieldset>
<legend>UserModels</legend>
@Html.HiddenFor(model => model.Id)
<div class="editor-label">
@Html.LabelFor(model => model.FirstName)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.FirstName)
@Html.ValidationMessageFor(model => model.FirstName)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.LastName)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.LastName)
@Html.ValidationMessageFor(model => model.LastName)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Address)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Address)
@Html.ValidationMessageFor(model => model.Address)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Email)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Email)
@Html.ValidationMessageFor(model => model.Email)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.DOB)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.DOB)
@Html.ValidationMessageFor(model => model.DOB)
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Salary)
</div>
<div class="editor-field">
@Html.EditorFor(model => model.Salary)
@Html.ValidationMessageFor(model => model.Salary)
</div>
<p>
<input type="submit" value="Save" />
</p>
</fieldset>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
|
此视图在我们的应用程序看起来如下:
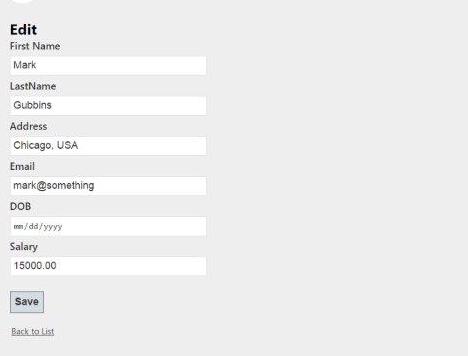
Delete.cshtml:
此视图将显示窗体用于删除现有用户。
@model AdvancedMVCApplication.Models.UserModels
@{
ViewBag.Title = "Delete";
}
<h2>Delete</h2>
<h3>Are you sure you want to delete this?</h3>
<fieldset>
<legend>UserModels</legend>
<div class="display-label">
@Html.DisplayNameFor(model => model.FirstName)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.FirstName)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.LastName)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.LastName)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Address)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Address)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Email)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Email)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.DOB)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.DOB)
</div>
<div class="display-label">
@Html.DisplayNameFor(model => model.Salary)
</div>
<div class="display-field">
@Html.DisplayFor(model => model.Salary)
</div>
</fieldset>
@using (Html.BeginForm()) {
@Html.AntiForgeryToken()
<p>
<input type="submit" value="Delete" /> |
@Html.ActionLink("Back to List", "Index")
</p>
} |
此视图在我们最终的应用程序看起来像这样:
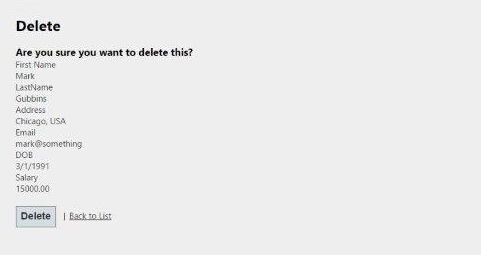
第6步:我们已经增加模型和视图在应用程序中。现在添加一个控制器,用于视图。 右键单击Controllers文件夹,然后点击 Add->Controller. 命名为: UserController.
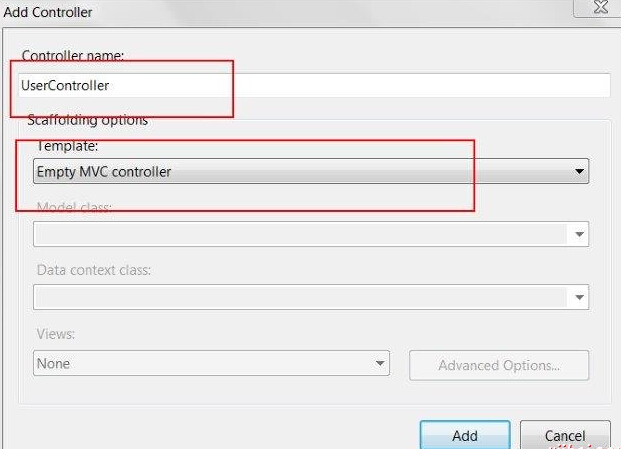
默认情况下,控制器类将用下面的代码来创建:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using AdvancedMVCApplication.Models;
namespace AdvancedMVCApplication.Controllers
{
public class UserController : Controller
{
private static Users _users = new Users();
public ActionResult Index()
{
return View(_users.UserList);
}
}
} |
在上面的代码中,Index方法将在呈现用户列表在Index页面上。
第6步:右键单击Index方法,并选择创建视图来创建Index页面视图(其中会列出所有用户,并提供选项来创建新的用户)。
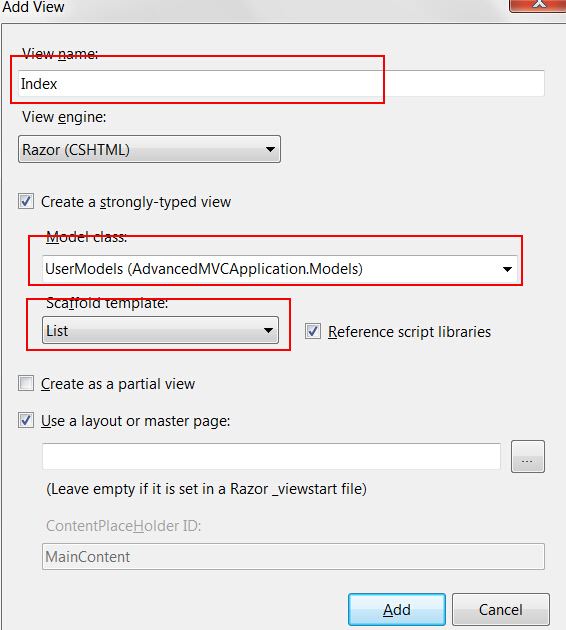
第7步:现在添加以下代码UserController.cs。在这段代码中,我们创建操作方法针对不同的用户操作,返回之前创建相应的视图。
我们将添加两个方法为每个操作:GET和POST。当获取数据时,其HttpGet被使用。HttpPost将用于创建/更新数据。例如,当我们要添加新用户,需要一个表单来添加用户,这是一个GET操作。当我们填写表格,并提交这些值,需要使用POST方法。
//Action for Index View
public ActionResult Index()
{
return View(_users.UserList);
}
//Action for UserAdd View
[HttpGet]
public ActionResult UserAdd()
{
return View();
}
[HttpPost]
public ActionResult UserAdd(UserModels userModel)
{
_users.CreateUser(userModel);
return View("Index", _users.UserList);
}
//Action for Details View
[HttpGet]
public ActionResult Details(int id)
{
return View(_users.UserList.FirstOrDefault(x => x.Id == id));
}
[HttpPost]
public ActionResult Details()
{
return View("Index", _users.UserList);
}
//Action for Edit View
[HttpGet]
public ActionResult Edit(int id)
{
return View(_users.UserList.FirstOrDefault(x=>x.Id==id));
}
[HttpPost]
public ActionResult Edit(UserModels userModel)
{
_users.UpdateUser(userModel);
return View("Index", _users.UserList);
}
//Action for Delete View
[HttpGet]
public ActionResult Delete(int id)
{
return View(_users.UserList.FirstOrDefault(x => x.Id == id));
}
[HttpPost]
public ActionResult Delete(UserModels userModel)
{
_users.DeleteUser(userModel);
return View("Index", _users.UserList);
} sers.UserList);
} |
第8步:最后要做的就是到App_Start文件夹找到RouteConfig.cs文件,并更改默认的控制器。
defaults: new { controller = "User", action = "Index", id = UrlParameter.Optional } |
第9步:下面是高级应用示例程序启动和运行。现在运行应用程序。将能够看到这样的应用程序,并可以执行添加,查看,编辑,删除用户,因为在前面的截图已经看到了所有功能。
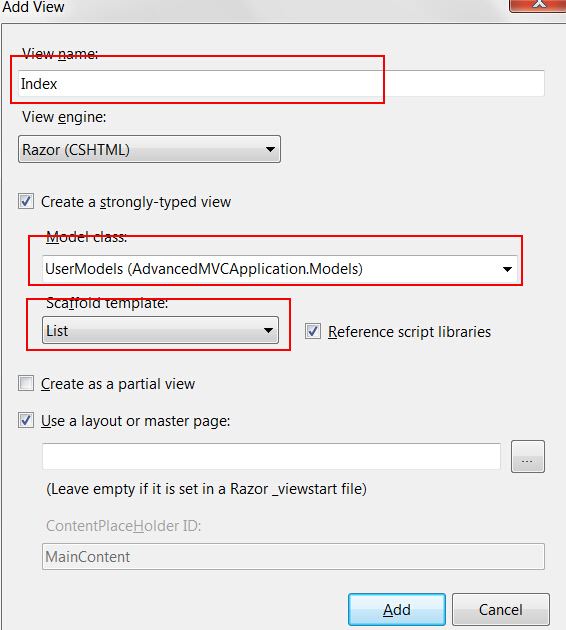
|